React is a popular JavaScript library for building user interfaces. One of its key features is the use of hooks, which were introduced in React 16.8. Hooks allow developers to use state and other React features in functional components, making it easier to manage complex UI logic. In this blog post, we’ll explore the world of hooks in React and learn how to use them effectively.
What Are Hooks?
Hooks are functions that allow you to “hook into” React state and lifecycle features from functional components. Prior to hooks, you could only use state and lifecycle methods in class components. Hooks provide a more intuitive way to work with these concepts in functional components.
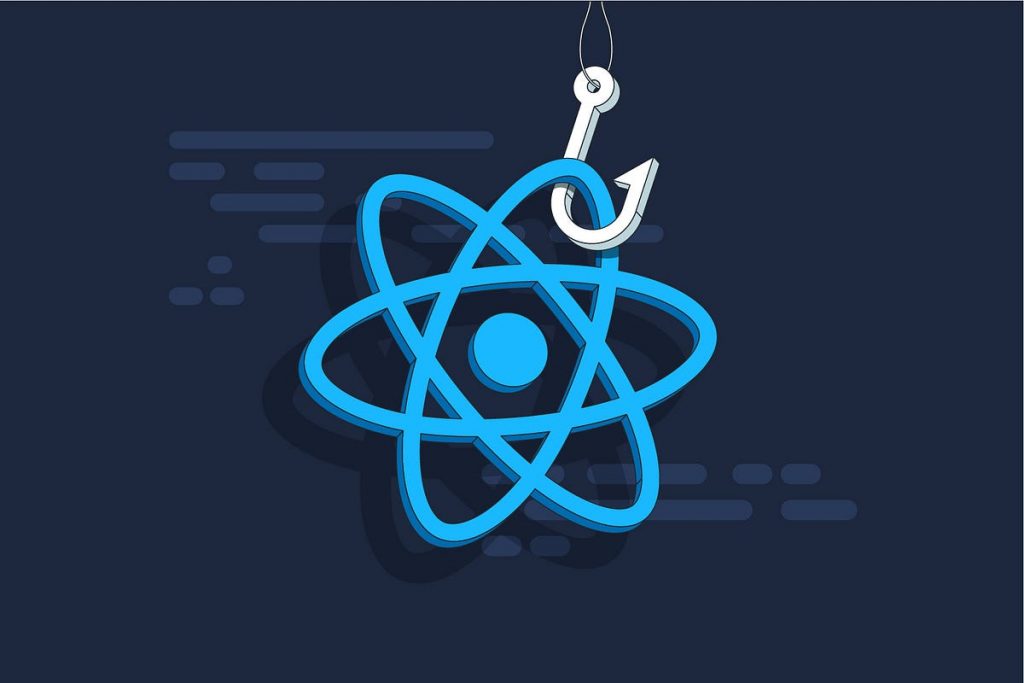
Commonly Used Hooks:-
React provides several built-in hooks that cover a wide range of use cases. Here are some of the most commonly used hooks:
1. useState: This hook allows you to add state to your functional components. It takes an initial state as an argument and returns the current state and a function to update it.
const[count , setCount] = useState(0);
2. useEffect: This hook lets you perform side effects in your components. It takes two arguments: a function to run the effect and an array of dependencies to control when the effect should run.
useEffect(()=> {
//side effect code here
},[dependency]);
3. useContext: This hook allows you to access the context of your application. It’s useful for sharing data between components without prop drilling.
const value = useContext(myContext);
4. useReducer: This hook is used for managing more complex state logic. It’s similar to `useState` but allows you to specify how the state should change based on actions.
const [state, dispatch] = useReducer(reducer, initialState);
5. useRef: This hook provides access to a mutable reference that persists across renders. It’s often used for accessing and managing DOM elements directly.
const myRef = useRef();
Rules of Using Hooks :-
To use hooks correctly, you should follow these rules:
1. Hooks should only be called at the top level of a function component or a custom hook. They should not be called inside loops, conditions, or nested functions.
2. Hooks should have the same order in every render. This ensures that React can correctly associate stateful values with the corresponding hooks.
3. Custom hooks should always start with the word “use.” This convention helps differentiate them from regular functions.
Creating Custom Hooks
In addition to the built-in hooks, you can create custom hooks to encapsulate and reuse stateful logic. Custom hooks are regular JavaScript functions that can call other hooks and hold their own state. Here’s an example of a custom hook that manages form input:
import { useState } from ‘react’;
function useFormInput(initialValue) {
const [value, setValue] = useState(initialValue);
const handleChange = (e) => {
setValue(e.target.value);
};
return {
value,
onChange: handleChange,
};
}
Benefits of Using Hooks :-
Using hooks in React offers several benefits:
1. Improved Code Reusability: Hooks make it easier to share and reuse stateful logic across components, reducing code duplication.
2. Easier to Understand: Functional components with hooks often have simpler and more predictable code compared to class components with lifecycle methods.
3. Better Performance: Hooks enable React to optimize component updates more effectively, potentially improving performance.
Conclusion :-
Hooks have become an integral part of React development, and mastering them is essential for building efficient and maintainable applications. In this blog post, we’ve covered the basics of hooks, commonly used hooks, rules for using them, and how to create custom hooks. With this knowledge, you can start building React applications that are more readable, reusable, and performant.
1 comment